Electronics Tutorial Website
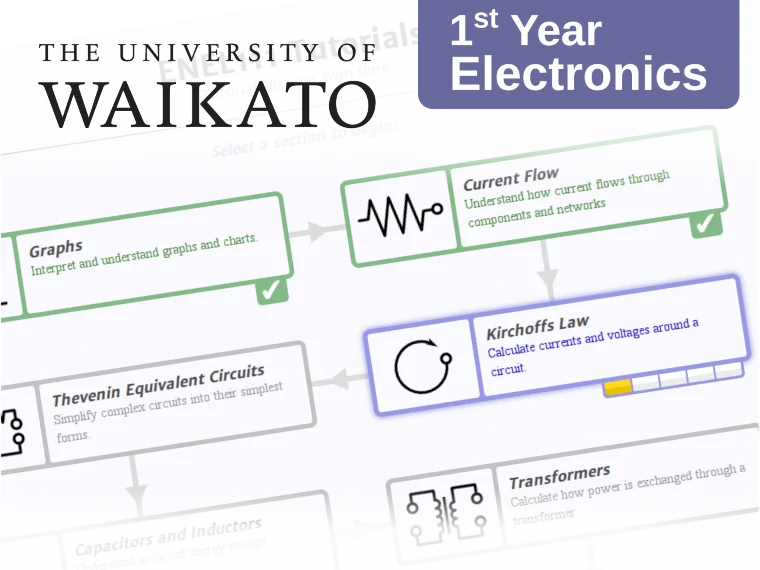
During my studies I helped to build an online tutorial website for first year students at The University of Waikato. My job was to revamp an existing site and turn it into something more professional. A large part of the code-base was rewritten, utilising libraries for page generation (PHP HTMLifier), restructured MySQL database, and creating a fresh looking front-end.
Before:
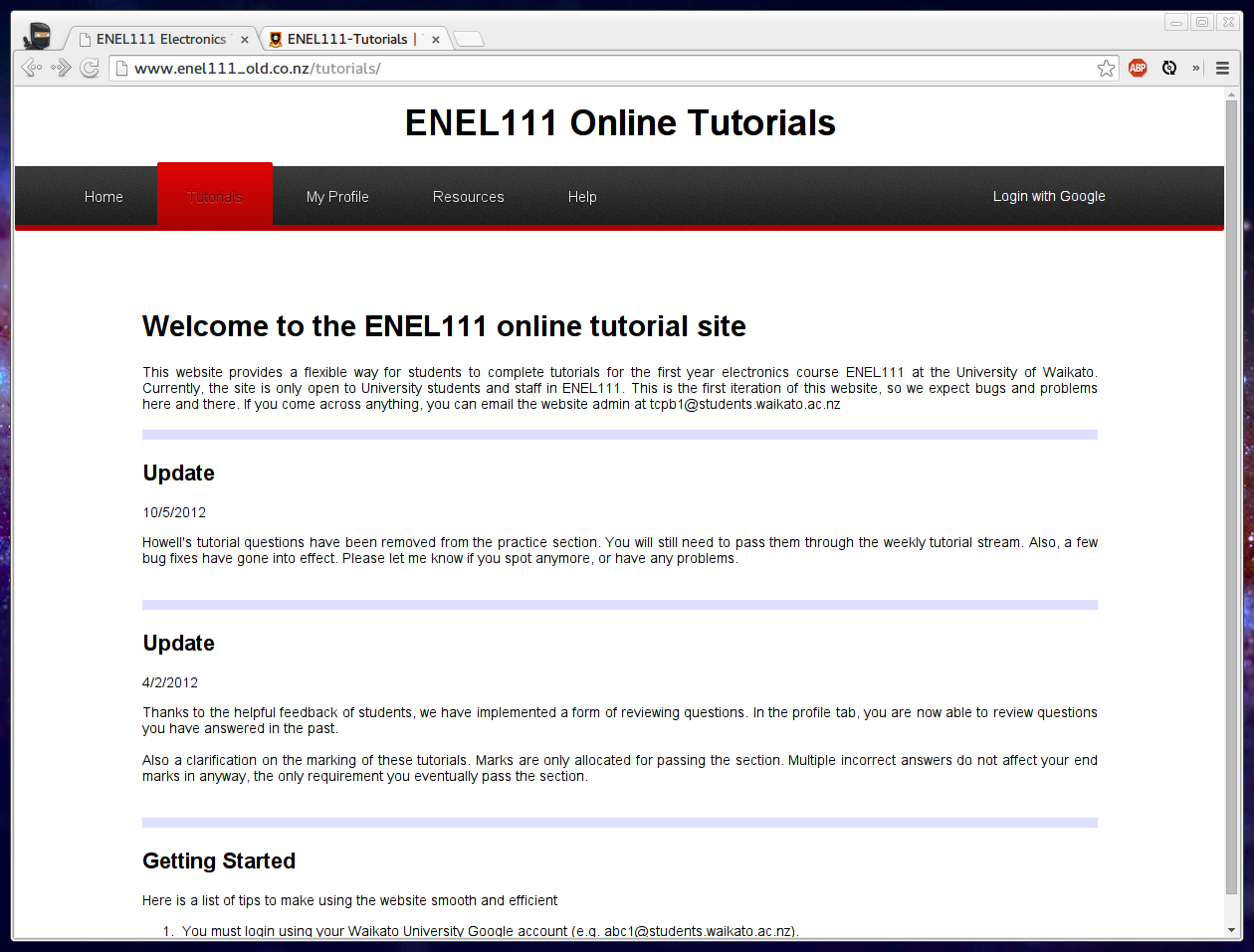
After:
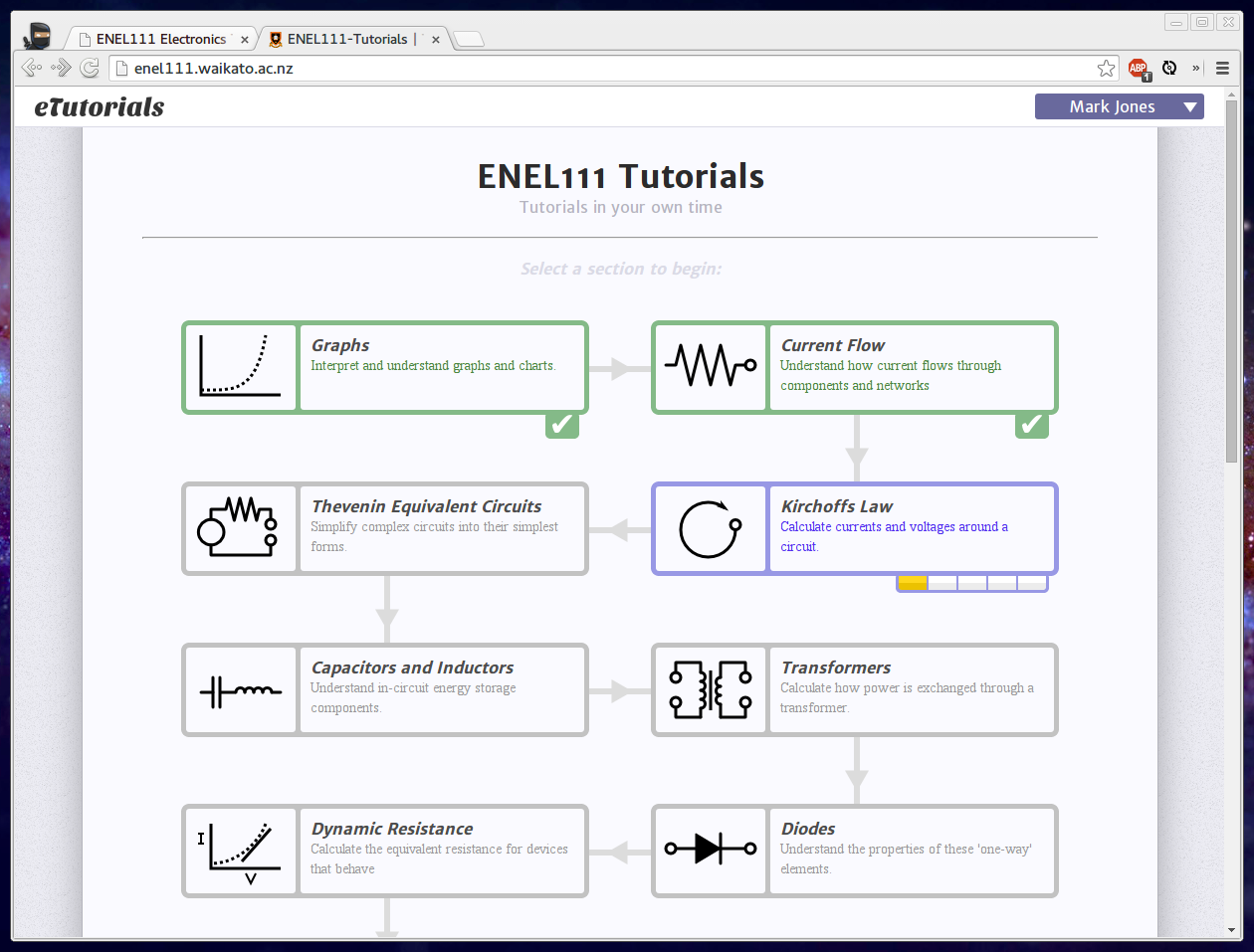
Answering questions:
Backend:
Almost all of the existing code was replaced. My HTML generation library for PHP (PHP-HTMLifier) was used throughout to generate pages. This library removes the need to spread echo statements throughout an otherwise HTML document, making the code much easier to read and maintain. The SQL database had redundant fields removed and inconsistent field names made consistent. New linker tables were added to remove data duplication and facilitate simpler updates.
One thing I really liked about Django was the way database tables are handled directly as Python classes. This makes things much simpler for the programmer because they don't have write a query to get a row from the database. Imitating this, I implemented wrappers around database tables that handled rows the same way. This made dealing with the database much cleaner and simpler.
The following code snippet gives an example of how compact the page generation logic has been made.
Note the
$section = new Section($sectionId)
line, which creates and fetches the relevant question from the database.
If fields within the object are changed during run-time, they are automatically saved to the database when the object is destroyed.
<?php
// This code creates the practise tutorial page.
//
// It loads our bootstrapping code, checks the client is logged in,
// retrieves any GET variables and parses them (with safety checks),
// checks the user is allowed to practise on this section,
// gets a suitable question for the client to practise with and
// renders the page using the PHP-HTMLifier renderer.
//
include(dirname($_SERVER['DOCUMENT_ROOT']).'/lib/functions.php');
$client->require_loggedIn();
$sectionId = getVar('get', 'section', 'int', True);
if ($client->current_section <= $sectionId) redirect('/messages/?msg=mustPass');
else $section = new Section($sectionId);
$question = $objectFactory->question_client_getNext_practise($client, $section);
$page = new BasePage($section->name, $client);
$page->append(View::question_header($section, $question));
$page->append(View::pose_question($question, True));
Course editor:
The site had to make it easy for lectures to add new content.
Usability improvements made here were
- Ability to navigate through all questions while in the editor (no need hit the back button).
- Asynchronous saving and image uploading to prevent interruptions while adding content.
- Automatic answer shuffling - so the content editor doesn't have to worry about correct answer position patterns.